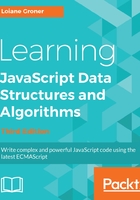
上QQ阅读APP看书,第一时间看更新
Variables scope with let and const
To understand how variables declared with the let or const keywords work, let's use the following example (you can run the example using the following URL: https://goo.gl/NbsVvg):
let movie = 'Lord of the Rings'; // {1} //var movie = 'Batman v Superman'; // error movie already declared function starWarsFan() { const movie = 'Star Wars'; // {2} return movie; } function marvelFan() { movie = 'The Avengers'; // {3} return movie; } function blizzardFan() { const isFan = true; let phrase = 'Warcraft'; // {4} console.log('Before if: ' + phrase); if (isFan) { let phrase = 'initial text'; // {5} phrase = 'For the Horde!'; // {6} console.log('Inside if: ' + phrase); } phrase = 'For the Alliance!'; // {7} console.log('After if: ' + phrase); } console.log(movie); // {8} console.log(starWarsFan()); // {9} console.log(marvelFan()); // {10} console.log(movie); // {11} blizzardFan(); // {12}
This will be the output from the previous code:
Lord of the Rings Star Wars The Avengers The Avengers Before if: Warcraft Inside if: For the Horde! After if: For the Alliance!
The following is an explanation of why we got this output:
- In line {1}, we declared a movie variable with the value Lord of the Rings, and we output its value in line {8}. This variable has a global scope, as you learned in the Variable scope section of this chapter.
- In line {9}, we executed the starWarsFan function. Inside this function, we also declared a variable named movie in line {2}. The output from this function is Star Wars because the variable from line {2} has a local scope, meaning it is only valid inside this function.
- In line {10}, we executed the marvelFan function. Inside this function, we changed the value of the movie variable (line {3}). This variable made a reference to the global variable declared in line {1}. Therefore, we got the output The Avengers in line {10} and in line {11}, where we output the global variable.
- Finally, we executed the blizzardFan function in line {12}. Inside this function, we declared a variable named phrase (line {4}) with the scope of the function. Then, in line {5}, again, we will declare a variable named phrase, but this time, this variable will only have a scope inside the if statement.
- In line {6}, we changed the value of phrase. As we are still inside the if statement, only the variable declared in line {5} would have its value changed.
- Then, in line {7}, we again changed the value of phrase, but as we are not inside the block of the if statement, the value of the variable declared in line {4} is changed.
This scope behavior is the same as in other programming languages, such as Java or C. However, this was only introduced in JavaScript through ES2015 (ES6).
Note that in the code presented in the section, we are mixing let and const. Which one should we use? Some developers (and also some lint tools) prefer using const if the reference of the variable does not change. However, this is a matter of personal preference; there is no wrong choice!