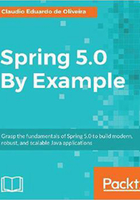
UserService
The UserService class is quite similar to the CategoryService, but the rules are about the User entity, for this entity we do not have anything special. We have the @Service annotation, and we received the UserRepository constructor as well. It is quite simple and easy to understand. We will show the UserService implementation, and it must be like this:
package springfive.cms.domain.service;
import java.util.List;
import java.util.UUID;
import org.springframework.stereotype.Service;
import springfive.cms.domain.models.User;
import springfive.cms.domain.repository.UserRepository;
import springfive.cms.domain.vo.UserRequest;
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User update(String id,UserRequest userRequest){
final User user = this.userRepository.findOne(id);
user.setIdentity(userRequest.getIdentity());
user.setName(userRequest.getName());
user.setRole(userRequest.getRole());
return this.userRepository.save(user);
}
public User create(UserRequest userRequest){
User user = new User();
user.setId(UUID.randomUUID().toString());
user.setIdentity(userRequest.getIdentity());
user.setName(userRequest.getName());
user.setRole(userRequest.getRole());
return this.userRepository.save(user);
}
public void delete(String id){
final User user = this.userRepository.findOne(id);
this.userRepository.delete(user);
}
public List<User> findAll(){
return this.userRepository.findAll();
}
public User findOne(String id){
return this.userRepository.findOne(id);
}
}
Pay attention to the class declaration with @Service annotation. This is a very common implementation in the Spring ecosystem. Also, we can find @Component, @Repository annotations. @Service and @Component are common for the service layer, and there is no difference in behaviors. The @Repository changes the behaviors a little bit because the frameworks will translate some exceptions on the data access layer.