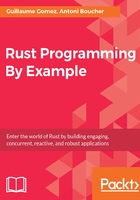
上QQ阅读APP看书,第一时间看更新
Tetris
Okay, we're now ready to start writing down our tetris!
First, let's fulfill our main.rs file in order to check whether everything is working as expected:
extern crate sdl2; use sdl2::pixels::Color; use sdl2::event::Event; use sdl2::keyboard::Keycode; use std::time::Duration;
use std::thread::sleep; pub fn main() { let sdl_context = sdl2::init().expect("SDL initialization
failed"); let video_subsystem = sdl_context.video().expect("Couldn't get
SDL video subsystem"); let window = video_subsystem.window("rust-sdl2 demo: Video", 800,
600) .position_centered() .opengl() .build() .expect("Failed to create window"); let mut canvas = window.into_canvas().build().expect("Failed to
convert window into canvas"); canvas.set_draw_color(Color::RGB(255, 0, 0)); canvas.clear(); canvas.present(); let mut event_pump = sdl_context.event_pump().expect("Failed to
get SDL event pump"); 'running: loop { for event in event_pump.poll_iter() { match event { Event::Quit { .. } | Event::KeyDown { keycode: Some(Keycode::Escape), .. } =>
{ break 'running }, _ => {} } } sleep(Duration::new(0, 1_000_000_000u32 / 60)); } }
You'll note the following line:
::std::thread::sleep(Duration::new(0, 1_000_000_000u32 / 60));
It allows you to avoid using all your computer CPU time needlessly and only rendering 60 times every second at most.
Now run the following in your terminal:
$ cargo run
If you have a window filled with red (just as shown in the following screenshot), then everything's fine!

Figure 2.4