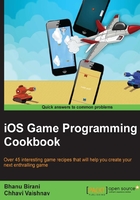
Anatomy of game projects
In this section we will see the basics of a game project. This includes understanding the basic architecture and work flow of game projects. Here we will learn about the scene and layers and their importance in games.
Getting ready
Complete game development is dependent on three core components: scenes, nodes, and sprites mentioned earlier. We need to have a command over these components to effectively start on game development.
How to do it...
Internally, the life cycle is executed as per the scenes—nodes are added and actions applied on these nodes. It also includes attaching some physics bodies to the nodes, support for cropping, applying animation and effects to all or a part of the content, detecting forces and collision, drawing in OpenGL, and many more things.
Apart from all this, there is an overridden update method in SKScene
, which is called for each frame of the game with the current time interval as a parameter. There you can add your actual game logic specifying what to do at what time and many more things as it is called by every frame that is rendered.
For an example, we can track the difference in time between the current time and the last updated time.
- As the current time interval is received in the update method, define the properties for difference in time and last updated time.
@property (nonatomic, assign) NSTimeInterval lastUpdatedTime; @property (nonatomic, assign) NSTimeInterval diffTime;
- Now calculate difference in time by subtracting the last updated time from the current time and updating the lastUpdatedTime to the current time.
self.diffTime = currentTime - self.lastUpdatedTime; self.lastUpdatedTime = currentTime;
- At last the update method looks like this:
- (void)update:(CFTimeInterval)currentTime { /* Called before each frame is rendered */ self.diffTime = currentTime - self.lastUpdatedTime; self.lastUpdatedTime = currentTime; }
Now this is the place where we are going add our maximum game logic—all the adding, removing, animating, updating all nodes, sprites, and actions will take place inside this method. We can also take the help of currentTime
to maintain some timers simply using float variables (updating them by the diffTime
and firing time events whenever required according to our game design or logic).
How it works...
All that we see running on the screen are just frames added by a time interval, which is driven by a SKScene
added as a child on the SKView
that serves as the main scene of the game.
As shown in the following diagram, a frame circle is present, which depicts the execution cycle of the game project for each frame:

Some of the methods from the preceding diagram are explained as follows:
- An update method of
SKScene
is called where we can add, remove, animate, and update different kinds of nodes and actions. SKScene
evaluates its actions that are running for the current frame following some life cycle calls such asdidEvaluateActions
.SKScene
has its own physics simulation, so if some bodies are added to it, the physics simulation is also evaluated such as collision detection, applying forces, and so on.- All the methods mentioned earlier contribute to the final rendering of SKView, which is displayed as a frame to the user. Hence, regular running of these frames makes the game appear as an environment.